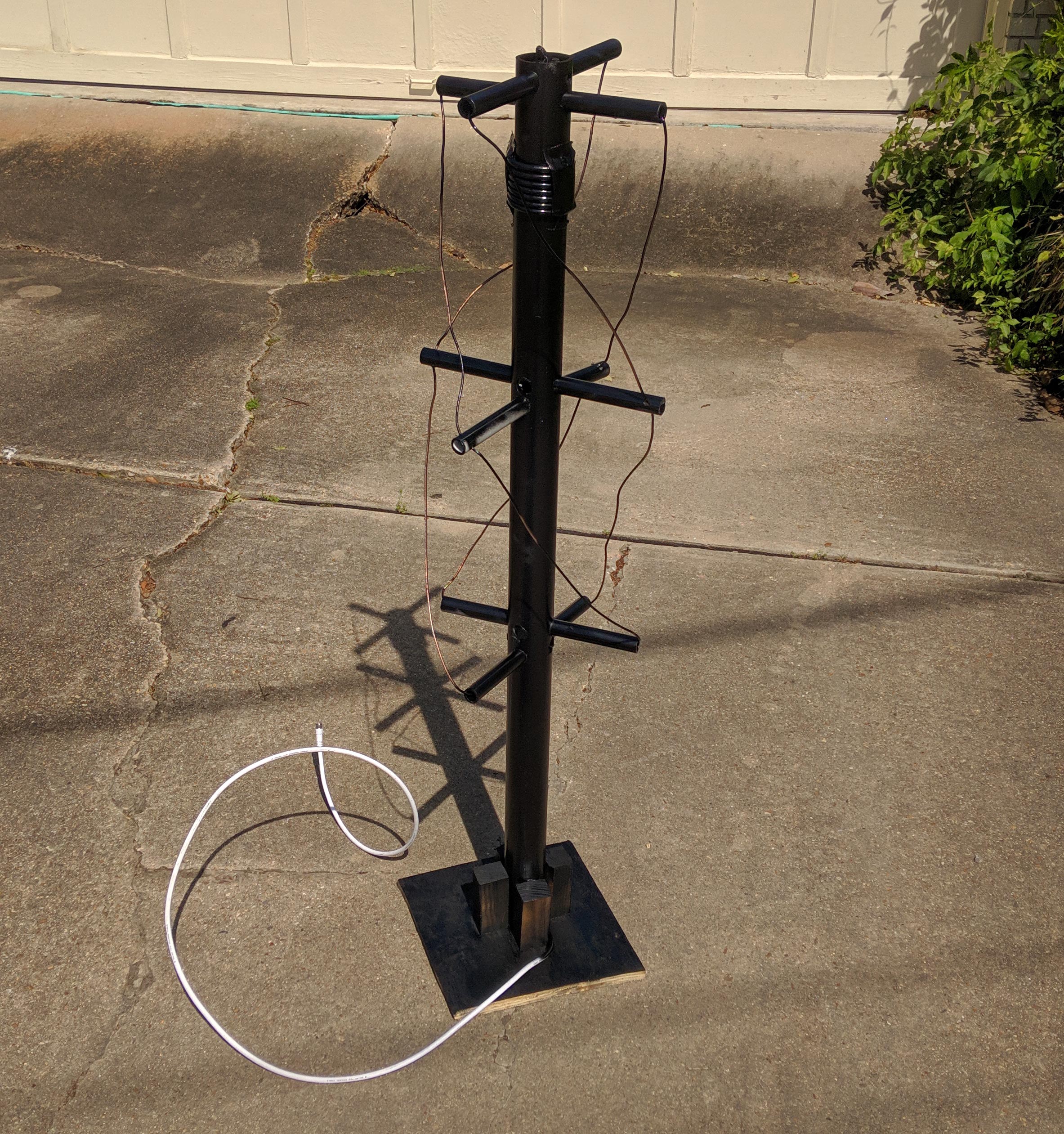
Decoding radio transmissions received by a consumer Software-Defined Radio
I used a 25 dollar software-defined radio connected to homemade antennas in order to receive radio transmissions from airplanes and satellites.
Learn More This all started with the crazy idea to make a vehicle using a treadmill motor. My justification was that a treadmill has a motor that can move a person so it should be able to move a person plus a couple pounds for a frame and wheels. Boy was I wrong.
The parts were really not that hard to take out of the treadmill; You just need a good drill or a lot of grip strength to unscrew the chassis. The motor was screwed to the chassis with a belt between it and the axle. The motor controller had a few electrical connections leading from the headpiece of the treadmill. There were also connections going to the motor, linear actuator, and a tachometer.
Using this schematic for my MC2100LS-30 Rev motor controller, I built a small circuit to activate the motor controller and start the motor. This same circuit was able to move the linear actuator as well.
Once I was able to drive the motor, I began building a frame for the go-kart out of 2x4s and wood nails. This is where the project fell apart. I could not find good wheels for the axle that I salvaged from the treadmill. Since I am not a mechanical engineer, I could not figure out another way to attach the wheels to the axle other than using hot glue. The wheels did not adhere to the metal and would slide around when the axle moved. I also could not keep tension on the belt which meant the motor could not move the axle.
Below is the code I used to control the motor controller. Keep in mind lots of this is commented out in order to test different aspects of my code. This is a working version that spins the motor as soon as it is connected. My full setup is on my github.
#include
//definitions
#define PWM_CYCLE 50.0
#define PWM_OUT 9
#define MAX_DUTY 869
#define MIN_DUTY 0
#define inPin 12
//int
int speedLevel;
int ACC = 0;
int reading;
int previous = LOW;
void setup()
{
//init pwmout and timer1
pinMode(PWM_OUT, OUTPUT);
pinMode(10, OUTPUT);
Timer1.initialize(PWM_CYCLE * 1000);
Timer1.pwm(PWM_OUT, 0);
//acc switch
pinMode(inPin, INPUT);
Serial.begin(9600);
}
We first set our output pins to OUTPUT. We also have to initialize Timer1 and start Timer1 with the output pin. Serial must also be turned on for logging.
void loop()
{
reading = digitalRead(inPin);
//if switch is in on position, accelerate
if (reading == HIGH && previous == LOW) {
ACC = ACC + 20;
}
//if switch is in off position, decelerate
if (reading == LOW && previous == HIGH) {
ACC = ACC - 20;
}
}
This portion of the code implements an on/off switch in lieu of a potentiometer. Most of the above code is commented out because I did not opt to include a potentiometer in this project.
speedLevel = map(ACC, 0, 1023, 0, MAX_DUTY);
Timer1.setPwmDuty(PWM_OUT, speedLevel);
Serial.print("speed: " + speedLevel);
Serial.print("ACC: " + ACC);
We need to begin sending PWM signals to the motor controller. We do this mapping our ACC variable (acceleration) to the MAX_DUTY of the motor. We then tell Timer1 to output a PWM signal with a duty of speedLevel (our map of ACC and MAX_DUTY). We also print the speed and acceleration for testing and debug purposes.
All in all, the project took a lot of time but was very fulfilling. I'll never forget the moment I saw the motor controller board LED turn on and having the motor turn. The project took about 2 months because I had quite a few issues when taking the treadmill apart and I could not find a good schematic for the motor controller. Only when I found that was I able to finish the project.
I used a 25 dollar software-defined radio connected to homemade antennas in order to receive radio transmissions from airplanes and satellites.
Learn MoreWith an arduino, I was able to control the salvaged linear actuator, motor, and motor controller of a broken treadmill. With this set up, I attempted to create a working go-kart.
Learn More